使用video.js视频播放器以及绘制声纹图
创建时间:
字数:4.1k
阅读:
本文介绍使用video.js实现视频播放器以及使用wavesurfer.js实现对声纹图的绘制。
video.js 是最强大的网页嵌入式 HTML 5 视频播放器的组件库之一,也是大多数人首选的网页视频播放解决方案。本文介绍使用video.js实现视频播放器以及使用wavesurfer.js实现对声纹图的绘制,如果你只需要视频播放不需要声纹图可直接跳过wavesurfer.js部分。
首先安装
1
| npm i video.js wavesurfer.js
|
一、视频播放器
HTML
1
| <video ref= "video" controls></video>
|
JS
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| import Videojs from 'video.js' import 'video.js/dist/video-js.css'
export default { data () { return { myPlayer: null, wavesurfer: null, } }, mounted () { this.initVideo() }, methods: { initVideo () { this.myPlayer = Videojs(this.$refs.video, { playbackRates: [0.5, 1.0, 1.5, 2.0], autoplay: true, muted: false, loop: false, preload: 'auto', language: 'zh-CN', aspectRatio: '16:9', fluid: true, sources: [{ type: 'video/mp4', src: 'src', }], poster: '', notSupportedMessage: '此视频暂无法播放,请稍后再试', controlBar: { timeDivider: true, durationDisplay: true, remainingTimeDisplay: true, fulscreenToggle: true, } }) } } }
|
video.js在原生video的基础上封装了很多功能,可以通过初始化的时候option来配置,更多功能可查看官网文档配置项:https://videojs.com/guides/options/
二、声纹图
HTML
1
| <div ref= "waveform" style= "pointer-events: none" ></div>
|
JS
引入
1
| import WaveSurfer from 'wavesurfer.js'
|
在上面 initVideo
方法中加上如下对声纹图初始化的代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| this.wavesurfer = WaveSurfer.create({ container: this.$refs.waveform, waveColor: 'violet', barWidth: 1, barHeight: 1.5, cursorColor: 'red', progressColor: 'blue', backend: 'MediaElement', height: 60, audioRate: '1', }) this.wavesurfer.load('src') this.wavesurfer.on('ready', () => { this.wavesurfer.setMute(true) }
|
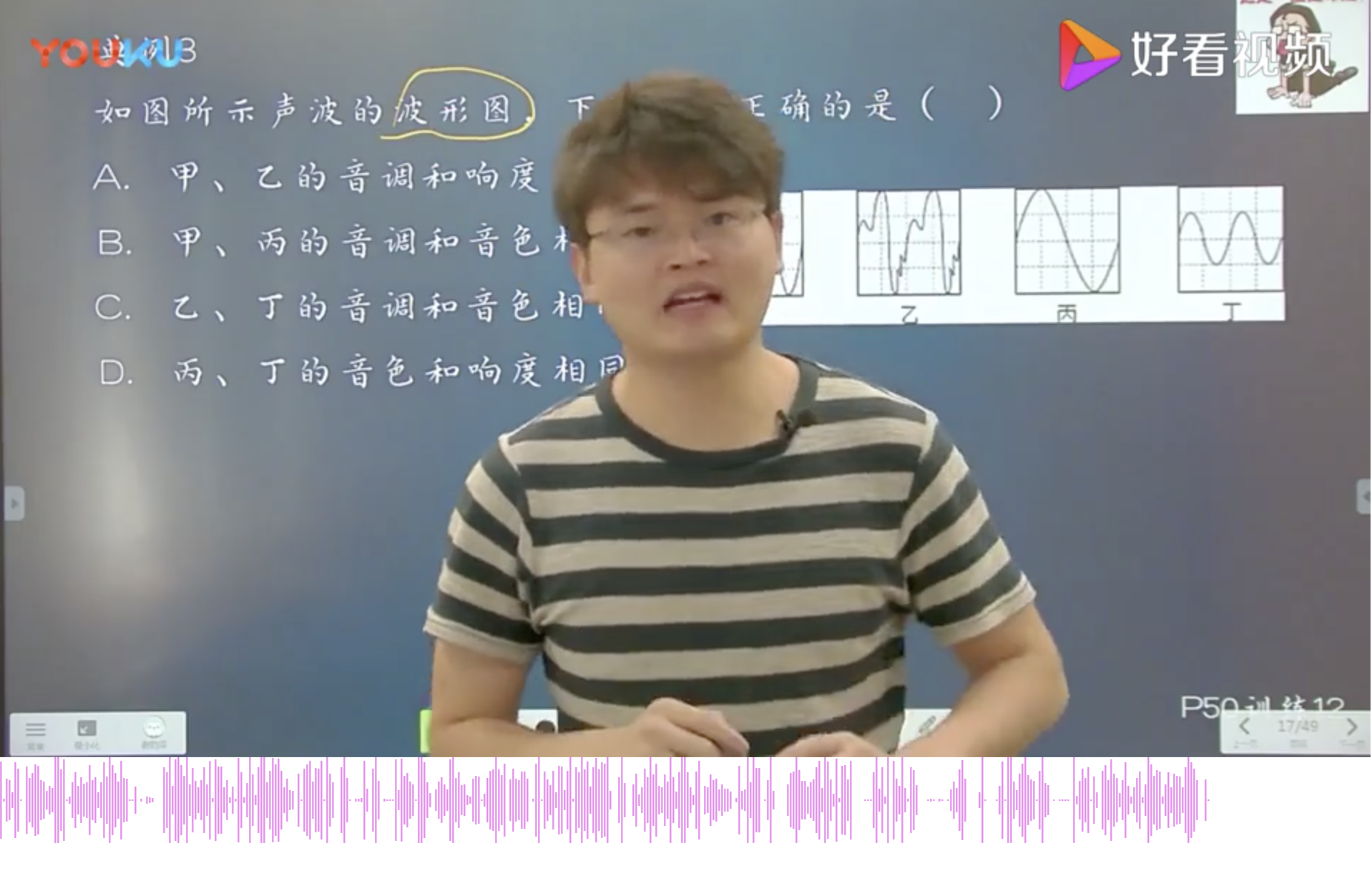
三、视频和声纹图同歩
当我们操作视频时,比如拖动进度条,切换倍速,那么声纹图也应该联动,所以我们需要给视频组件加上对应事件从而控制声纹图,下面贴出联动的代码
HTML
1 2 3 4 5 6 7 8 9
| <video ref= "video" controls @seeked="seeked($event)" @ratechange = "onRate($event)" @pause = "onPause($event)" @play= "onPlay($event)" @ended = "onPlayerEnded($event)" ></video>
|
JS
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| onRate (i) { this.wavesurfer.setPlaybackRate(i.path[0].playbackRate) },
seeked (i) { this.wavesurfer.seekTo(this.myPlayer.currentTime() / this.myPlayer.duration()) },
onPause(){ this.wavesurfer.pause() },
onPlay(){ this.wavesurfer.play() },
onPlayerEnded($event) { console.log($event) },
|
四、附录1:video.js 常用功能
1. 配置项option
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| autoplay: false, controls: true, width: 300, height: 300, loop: false, muted: false, poster: '', src: '', techOrder: ['html5', 'flash'], notSupportedMessage: false, plugins: {}, sources: [{src: '//path/to/video.mp4', type: 'video/mp4'}], aspectRatio: '16:9', fluid: false, preload: "metadata",
|
2. 事件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| this.myPlayer.on('suspend', function() { console.log("延迟下载") }); this.myPlayer.on('loadstart', function() { console.log("客户端开始请求数据") }); this.myPlayer.on('progress', function() { console.log("客户端正在请求数据") }); this.myPlayer.on('abort', function() { console.log("客户端主动终止下载") }); this.myPlayer.on('error', function() { console.log("请求数据时遇到错误") }); this.myPlayer.on('stalled', function() { console.log("网速失速") }); this.myPlayer.on('play', function() { console.log("开始播放") }); this.myPlayer.on('pause', function() { console.log("暂停") }); this.myPlayer.on('loadedmetadata', function() { console.log("成功获取资源长度") }); this.myPlayer.on('loadeddata', function() { console.log("渲染播放画面") }); this.myPlayer.on('waiting', function() { console.log("等待数据") }); this.myPlayer.on('playing', function() { console.log("开始回放") }); this.myPlayer.on('canplay', function() { console.log("可以播放,但中途可能因为加载而暂停") }); this.myPlayer.on('canplaythrough', function() { console.log("可以播放,歌曲全部加载完毕") }); this.myPlayer.on('seeking', function() { console.log("寻找中") }); this.myPlayer.on('seeked', function() { console.log("寻找完毕") }); this.myPlayer.on('timeupdate', function() { console.log("播放时间改变") }); this.myPlayer.on('ended', function() { console.log("播放结束") }); this.myPlayer.on('ratechange', function() { console.log("播放速率改变") }); this.myPlayer.on('durationchange', function() { console.log("资源长度改变") }); this.myPlayer.on('volumechange', function() { console.log("音量改变") });
|
3.常用方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| const whereYouAt = this.myPlayer.currentTime() const howLongIsThis = this.myPlayer.duration() const whatHasBeenBuffered = this.myPlayer.buffered() const howMuchIsDownloaded = this.myPlayer.bufferedPercent() const howLoudIsIt = this.myPlayer.volume() const howWideIsIt = this.myPlayer.width() const howTallIsIt = this.myPlayer.height() this.myPlayer.play() this.myPlayer.pause() this.myPlayer.currentTime(120) this.myPlayer.volume(0.5) this.myPlayer.width(640) this.myPlayer.height(480) this.myPlayer.size(640,480) this.myPlayer.enterFullScreen() this.myPlayer.enterFullScreen()
|
4. 网络状态
1 2 3 4 5 6 7
| this.myPlayer.currentSrc this.myPlayer.src = value this.myPlayer.canPlayType(type) this.myPlayer.networkState this.myPlayer.load() this.myPlayer.buffered this.myPlayer.preload
|
5. 播放状态
1 2 3 4 5 6 7 8 9 10 11
| this.myPlayer.currentTime = value this.myPlayer.startTime this.myPlayer.duration this.myPlayer.paused this.myPlayer.defaultPlaybackRate = value this.myPlayer.playbackRate = value this.myPlayer.played this.myPlayer.seekable this.myPlayer.ended this.myPlayer.autoPlay this.myPlayer.loop
|
6. 视频控制
1 2 3
| this.myPlayer.controls this.myPlayer.volume = value this.myPlayer.muted = value
|
五、附录2:wavesurfer.js 常用功能
1. option配置项
- container:必填,可以是唯一的css3选择器,也可以是DOM元素
- scrollParent:true/false,要使波形滚动。
- audioRate:播放音频的速度。数字越小越慢。
- backgroundColor:更改波形容器的背景颜色。
- barGap:波浪条之间的可选间距(如果未提供)将以旧格式计算。
- barHeight:波形条的高度。大于 1 的数字将增加波形条的高度。
- barMinHeight:绘制波形条的最小高度。默认行为是在静音期间不绘制条形图。
- barRadius:使条形变圆的半径。
- barWidth:波的每一条线宽度
- cursorColor:指示播放头位置的光标填充颜色。
- cursorWidth:指示的宽度
- forceDecode:缩放时使用网络音频强制解码音频以获得更详细的波形。
- height:波形的高度。以像素为单位。
- hideScrollbar:是否在正常显示时隐藏水平滚动条。
- hideCursor:将鼠标悬停在波形上时隐藏鼠标光标。默认情况下会显示。
- interact:是否在初始化时启用鼠标交互。您可以在以后随时切换此参数。
- loopSelection:(与区域插件一起使用)启用所选区域的循环。
- maxCanvasWidth:单个画布的最大宽度(以像素为单位),不包括小的重叠(2 * pixelRatio,四舍五入到下一个偶数整数)。如果波形比此值长,则将使用额外的画布来渲染波形,这对于浏览器无法在单个画布上绘制的非常大的波形非常有用。该参数仅适用于MultiCanvas渲染器。
- mediaControls:这将启用媒体元素的本机控件。
- mediaType:
audio
或 video
。
- minPxPerSec:每秒音频的最小像素数。
- partialRender:使用 PeakCache 提高大波形的渲染速度。
- progressColor:光标后面波形部分的填充颜色。当progressColor和waveColor相同时,根本不会渲染进度波
- waveColor:光标后波形的填充颜色
- responsive:如果设置为true调整波形大小,则在调整窗口大小时。默认情况下,这是使用 100 毫秒超时去抖动的。如果此参数是一个数字,则表示该超时。
2.常用方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| this.wavesurfer.cancelAjax() this.wavesurfer.destroy() this.wavesurfer.empty() this.wavesurfer.getActivePlugins() this.wavesurfer.getBackgroundColor() this.wavesurfer.getCurrentTime() this.wavesurfer.getCursorColor() this.wavesurfer.getDuration() this.wavesurfer.getPlaybackRate() this.wavesurfer.getProgressColor() this.wavesurfer.getVolume() this.wavesurfer.getMute() this.wavesurfer.getFilters() this.wavesurfer.getWaveColor() this.wavesurfer.exportPCM(length, accuracy, noWindow, start) this.wavesurfer.exportImage(format, quality, type) this.wavesurfer.isPlaying() this.wavesurfer.load(url, peaks, preload) this.wavesurfer.loadBlob(url) this.wavesurfer.on(eventName, callback) this.wavesurfer.un(eventName, callback) this.wavesurfer.unAll() this.wavesurfer.pause() this.wavesurfer.play([start[, end]]) this.wavesurfer.playPause() this.wavesurfer.seekAndCenter(progress) this.wavesurfer.seekTo(progress) this.wavesurfer.setBackgroundColor(color) this.wavesurfer.setCursorColor(color) this.wavesurfer.setHeight(height) this.wavesurfer.setFilter(filters) this.wavesurfer.setPlaybackRate(rate) this.wavesurfer.setPlayEnd(position) this.wavesurfer.setVolume(newVolume) this.wavesurfer.setMute(mute) this.wavesurfer.setProgressColor(color) this.wavesurfer.setWaveColor(color) this.wavesurfer.skip(offset) this.wavesurfer.skipBackward() this.wavesurfer.skipForward() this.wavesurfer.setSinkId(deviceId) this.wavesurfer.stop() this.wavesurfer.toggleMute() this.wavesurfer.toggleInteraction() this.wavesurfer.toggleScroll() this.wavesurfer.zoom(pxPerSec)
|
3. 常用事件
使用on()和un() 方法订阅和取消订阅各种播放器事件
- audioprocess– 在音频播放时持续触发。也在寻找上火。
- dblclick – 双击实例时。
- destroy – 当实例被销毁时。
- error– 发生错误。回调将收到(字符串)错误信息。
- finish – 当它完成播放时。
- interaction – 与波形交互时。
- loading– 使用 fetch 或 drag’n’drop 加载时连续触发。回调将以百分比 [0…100] 接收(整数)加载进度。
- mute– 静音更改。回调将收到(布尔值)新的静音状态。
- pause – 音频暂停时。
- play – 播放开始时。
- ready– 加载音频、解码并绘制波形时。使用 MediaElement 时,这会在绘制波形之前触发,请参阅waveform-ready。
- scroll- 当滚动条移动时。回调将接收一个ScrollEvent对象。
- seek– 在寻求。回调将收到(浮动)进度[0…1]。
- volume– 关于音量变化。回调将接收(整数)新卷。
- waveform-ready– 在使用 MediaElement 后端绘制波形后触发。如果您使用的是 WebAudio 后端,则可以使用ready.
- zoom– 关于缩放。回调将接收(整数)minPxPerSec。
代码不是万能的,但不写代码是万万不能的
Dary记
-
更多干货,尽在公众号
转载请注明来源,文末有原始链接。欢迎对文章中的引用来源进行考证,欢迎指出任何有错误或不够清晰的表达。可以在下面评论区评论,也可以邮件至 dary1112@foxmail.com
文章标题:使用video.js视频播放器以及绘制声纹图
文章字数:4.2k
本文作者:Dary
发布时间:2023-01-06, 18:11:00
最后更新:2023-01-06, 19:32:21
原始链接:http://www.xiongdalin.com/2023/01/06/videojs/
版权声明: "署名-非商用-相同方式共享 4.0" 转载请保留原文链接及作者。